UV Sensor CircuitPython Library
Description of CircuitPython library for AS3771 UV sensor board
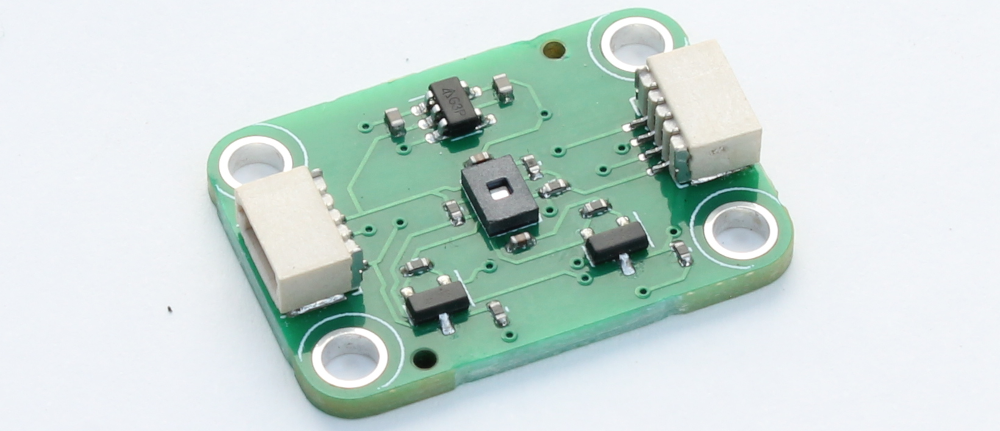
In our previous post, we shared the design for the AS7331 UV light sensor. In this post we describe a new CircuitPython library for communicating with the UV sensor.
iorodeo-as7331 CircuitPython Library
We developed the iorodeo-as7331 Circuitpython library to enable users to quickly get started using the UV sensor. The library is available on GitHub via the link below.
The library implements an I2C interface with the sensor and is based on Adafruit's I2CDevice helper class from the adafruit_bus_device library. The adafruit_bus_device library is the only required library.
Using the library
- Install iorodeo_as7331.py from the iorodeo_as7331 library and the adafruit_bus_device library on your device's CIRCUITPY drive.
- Import both the board module and the iorodeo_as7331 library itself. The board module is required for access to the devices I2C bus.
import board
import iorodeo_as7331 as as7331
- Next, get the
busio.I2C
object for the board’s I2C bus and then create a A7331 device object.
i2c = board.I2C()
sensor = as7331.AS7331(i2c)
- The irradiance responsivity of the sensor can be adjusted by setting the gain and integration_times for the sensor.
sensor.gain = as7331.GAIN_512X
sensor.integration_time = as7331.INTEGRATION_TIME_128MS
There are 12 allowed gain values which range from 1X up to 2048X in powers of 2. So the a allowed gains are 1X, 2X, 4X, 8X, 16X, 32X ..., etc.
There are 15 allowed integration_times which range from 1ms up to 16384ms in powers of 2. Thus the allowed integration_times are 1ms, 2ms, 4ms, 8ms, 16ms, ..., etc.
The irradiance values measured by the UV A, B, and C channels of the sensor and the temperature of the sensor can be obtained from the "values" property of the sensor.
uva, uvb, uvc, temp = sensor.values
The UV A, B and C irradiance values have units of μW/cm² and the temperature has units of degrees Celsius.
Putting this all together we have the following CircuitPython program for reading values from the sensor.
import time
import board
import iorodeo_as7331 as as7331
i2c = board.I2C()
sensor = as7331.AS7331(i2c)
sensor.gain = as7331.GAIN_512X
sensor.integration_time = as7331.INTEGRATION_TIME_128MS
while True:
uva, uvb, uvc, temp = sensor.values
print(f'uva: {uva:1.2f}, uvb: {uvb:1.2f}, uvc: {uvc:1.2f}, temp: {temp:1.2f}')
The AS7331 UV light sensor has three different measurement modes: "command",
"continuous" and "synchronous". The iorodeo-as7331 library currently only supports the "command" measurement mode. The two other measurement modes, "continuous" and "synchronous" could probably be added with a bit of effort.
For further details of the iorodeo_as7331 library checkout the examples in the github repository.
Purchase the AS7331 UV Sensor Board
We currently have a limited quantity of AS7331 sensor boards available in our online store. We are also finishing the firmware for implementing the sensor with our Open Colorimeter which we will be sharing in the next post.
Comments ()